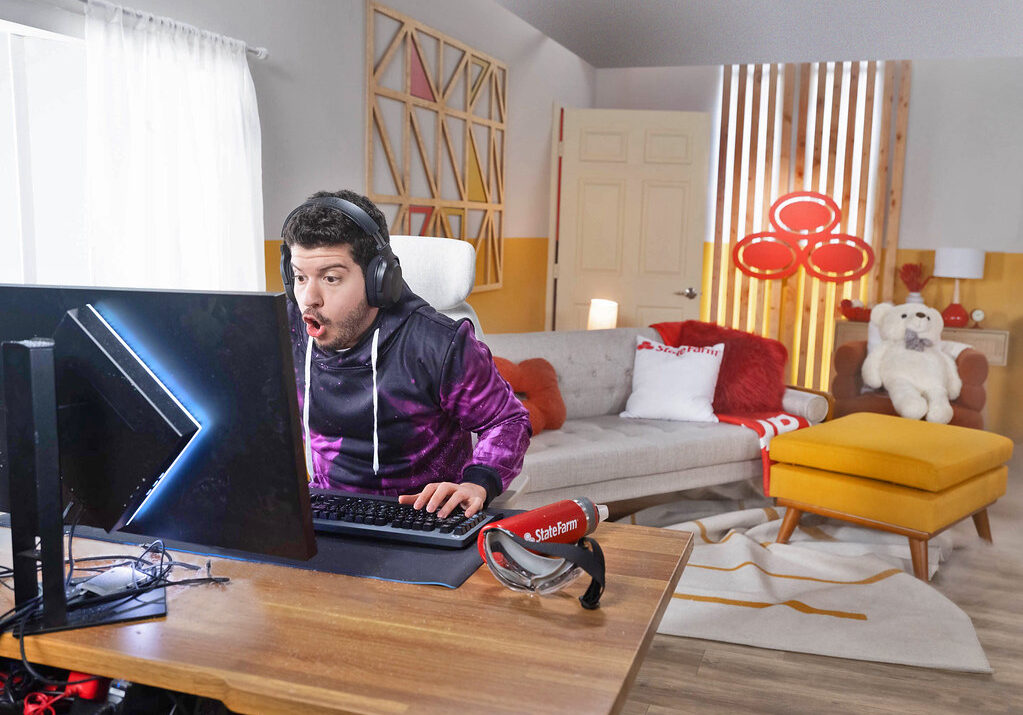
Twitch, the world’s foremost platform for live streaming, is a treasure trove of captivating content, ranging from epic gaming sessions to creative art showcases. While watching live streams is thrilling, there are times when you might want to save Twitch VODs (Video On Demand) for offline enjoyment. If you’re a Python enthusiast, scripting a solution is an excellent way to download Twitch VODs with ease. In this article, we’ll guide you through the process of using Python to automate the downloading of Twitch VODs, giving you a streamlined and customizable method for accessing your favorite content.
Contents
The Allure of Twitch VODs
Twitch VODs are recordings of past live streams, capturing both the video content and the chat interactions. These VODs offer numerous benefits:
- Convenience: VODs allow you to watch your favorite streams at your convenience, ensuring you don’t miss out on valuable content.
- Offline Viewing: Downloading VODs permits you to enjoy your preferred content offline, which is particularly useful when you’re on the move or have limited internet access.
- Archiving: For content creators, VODs serve as an archive, helping them preserve their past streams for future reference.
- Community Interaction: The chat interactions captured in VODs enable viewers to experience the community engagement that occurred during the live stream.
By using Python to script the download process, you can automate the retrieval of Twitch VODs according to your specific preferences.
Prerequisites: Python and Required Libraries
Before we proceed with scripting the download of Twitch VODs, you’ll need to ensure you have Python installed on your computer. You can download Python from the official Python website (https://www.python.org/downloads/).
Additionally, you’ll need to install the following Python libraries:
- requests: To make HTTP requests to Twitch.
- pytube: To download the video.
- twitch-python: To interact with Twitch’s API.
You can install these libraries using pip, the Python package manager. Run the following commands in your terminal or command prompt:
pip install requests
pip install pytube
pip install twitch-python
With Python and the necessary libraries in place, you’re ready to script the download of Twitch VODs.
Step-by-Step Guide to Download Twitch VODs with Python
Now, let’s walk through the process of using Python to download Twitch VODs:
Step 1: Import the Required Libraries
Start by importing the required Python libraries in your script. This includes requests, pytube, and twitch-python.
pythonCopy code
import requests from pytube import YouTube from twitch import TwitchClient
Step 2: Get Twitch API Credentials
To interact with Twitch’s API, you’ll need to obtain API credentials from the Twitch Developer portal. You can create an application and get your credentials at https://dev.twitch.tv/console/apps.
Step 3: Initialize the Twitch Client
Initialize the Twitch client with your API credentials.
pythonCopy code
twitch_client = TwitchClient(client_id='your_client_id', oauth_token='your_oauth_token')
Step 4: Get the VOD Information
You’ll need to obtain information about the Twitch VOD you want to download. This includes the VOD’s ID, which you can find from the VOD’s URL.
pythonCopy code
vod_url = 'https://www.twitch.tv/videos/your_vod_id' vod_id = vod_url.split('/')[-1] # Extract the VOD ID from the URL
Step 5: Get the VOD Details
Use the Twitch API to get information about the VOD.
pythonCopy code
vod = twitch_client.vods.get(vod_id) vod_url = vod['url'] vod_title = vod['title']
Step 6: Download the VOD
Use the pytube library to download the Twitch VOD. You can specify the download location and file format.
pythonCopy code
yt = YouTube(vod_url) yt.streams.filter(progressive=True, file_extension='mp4').first().download(output_path='download_path', filename=vod_title)
Replace 'download_path'
with the path where you want to save the VOD and customize the filename according to your preference.
Step 7: Script the Download Process
Combine the above steps into a Python script that you can run to download Twitch VODs. Make sure to include error handling and proper organization of your code.
import requests
from pytube import YouTube
from twitch import TwitchClient
# Initialize the Twitch client with your API credentials
twitch_client = TwitchClient(client_id='your_client_id', oauth_token='your_oauth_token')
# Extract the VOD ID from the URL
vod_url = 'https://www.twitch.tv/videos/your_vod_id'
vod_id = vod_url.split('/')[-1]
# Get information about the VOD
vod = twitch_client.vods.get(vod_id)
vod_url = vod['url']
vod_title = vod['title']
# Download the VOD with pytube
yt = YouTube(vod_url)
yt.streams.filter(progressive=True, file_extension='mp4').first().download(output_path='download_path', filename=vod_title)
Step 8: Execute the Script
Run your Python script to download the Twitch VOD. The script will extract the VOD details, access the VOD’s URL, and download it to your specified location.
Frequently Asked Questions
1. Is it legal to download Twitch VODs with Python for personal use?
Downloading Twitch VODs for personal use is generally considered legal, as long as you respect the rights of content creators and adhere to Twitch’s terms of service.
2. Can I download VODs with Python from any Twitch channel?
You can use Python to download VODs from any Twitch channel, as long as you have access to the VOD’s URL.
3. Are there any risks associated with using Python for downloading VODs?
Using Python for downloading VODs is generally safe. However, ensure that you respect copyright and content ownership and use the script responsibly.
4. Can I use Python to download VODs from a mobile device?
Python scripts are typically run on computers, but you can run Python on mobile devices with a suitable IDE or code editor and use the same script.
5. How can I support content creators on Twitch?
Supporting content creators on Twitch can be done through subscriptions, donations, or by watching ads during their live streams. These methods allow you to show appreciation for their content and support their work.
Conclusion
Scripting the download of VOD Download with Python offers a customized and efficient method for accessing your favorite content. By following the steps outlined in this guide and creating a Python script, you can automate the process of downloading Twitch VODs according to your specific preferences.
This method enables you to have control over the format and quality of your downloads, ensuring that you can enjoy your favorite Twitch content offline and at your convenience. Just remember to use this method responsibly, respecting the rights of content creators and adhering to Twitch’s terms of service as you continue to relish your downloaded VODs.